10th Computer Science Chapter 2 Programming in C
“Introduction to C Programming“
10th Computer Science Chapter 2 Programming in C introduces students to the fundamentals of programming using the C programming language. This chapter aims to provide a comprehensive understanding of basic programming constructs, syntax, and principles, laying a strong foundation for further exploration in computer science and software development. Some important keypoints of 10th Computer Science Chapter 2 Programming in C are given below.
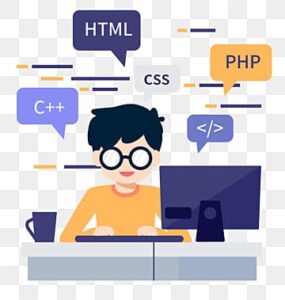
Key Topics Covered:
-
Introduction to Programming:
- Definition and significance of programming.
- Basic concepts of programming languages.
- Understanding the role of algorithms in programming.
-
Getting Started with C:
- History and significance of the C programming language.
- Setting up the development environment (e.g., IDEs, compilers).
- Writing and executing a simple C program.
-
C+Programming
-
Syntax and Structure of C Programs:
- Overview of C syntax rules.
- Structure of a C program (e.g., main function, comments, data types).
- Variables and constants in C.
-
Basic Input and Output Operations:
- Reading input from the user (scanf function).
- Displaying output to the user (printf function).
-
input output operations
-
Operators and Expressions:
- Arithmetic, relational, logical, and assignment operators.
- Precedence and associativity of operators.
- Understanding expressions and their evaluation.
-
Control Structures:
- Introduction to control structures: sequence, selection, and iteration.
- Conditional statements (if-else).
- Looping constructs (while, for loops).
-
Functions:
- Definition and significance of functions.
- Writing and calling functions in C.
- Function parameters and return values.
-
Arrays:
- Introduction to arrays and their importance.
- Declaration, initialization, and accessing elements of an array.
- Array manipulation and common operations.
-
Strings:
- Basics of strings in C.
- String manipulation functions (e.g., strlen, strcpy, strcat).
- Handling strings in C programs.
-
Pointers:
- Understanding pointers and their significance.
- Declaration and initialization of pointers.
- Pointer arithmetic and its applications.
Learning Outcomes: By the end of Chapter 2, students are expected to:
- Have a solid understanding of fundamental programming concepts and constructs.
- Be able to write, compile, and execute simple C programs.
- Demonstrate proficiency in using variables, control structures, functions, arrays, strings, and pointers in C programming.
- Apply problem-solving skills to solve basic programming challenges using C.
10th Computer Science Chapter 2 Programming in C serves as a crucial stepping stone for students as they delve deeper into the world of programming, preparing them for more advanced topics in computer science and software development.